A smooth life implementation based on https://arxiv.org/abs/1111.1567.
https://github.com/MartinPrograms/SmoothLife
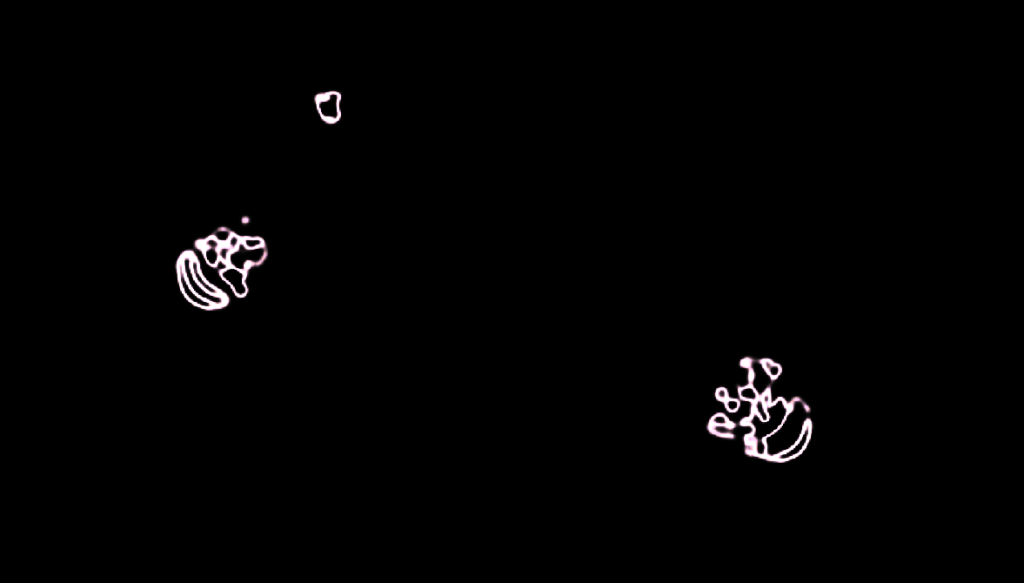
What is smooth life? well to understand smooth life we first need to understand Conway’s Game of Life.
Conway’s Game of Life (gol) is a simple cellular automata game, where every pixel (cell) has 2 states, dead, or alive, and depending on the state of the cells around it, it either stays alive, becomes alive or dies.
In gol’s case the rules are quite simple.
- A live cell dies if it has fewer than two live neighbors.
- A live cell with two or three live neighbors lives on to the next generation.
- A live cell with more than three live neighbors dies.
- A dead cell will be brought back to live if it has exactly three live neighbors.
From these 4 simple rules we get some great results without any complicated math!
You can check out more info about gol on this website! https://playgameoflife.com/
But what is the connection between gol and smooth life? Well, a lot! The only difference are the rules, and how the neighbours are sampled, unlike gol where we sample direct neighbours, we sample everything in a circular radius, with a weight attached to each point in that circle!
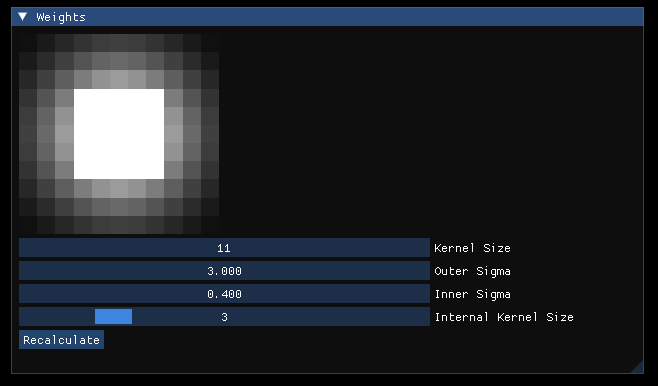
Saving the weights to a texture (you see above) saves on a lot of calculations, and replaces it with a memory read which is much more efficient than calculating the weights per pixel per loop.
float growth(float u0, float u1) {
float s = fastSigmoid(u0, 0.5, sig);
float t1 = t1au * u0 + t1bu;
float t2 = t2au * u0 + t2bu;
return s * (1.0 - s) * (s_u1u * u1 + (1.0 - s_u1u) * (t1 - t2));
}
After we sample the weights of our neighbours and normalising those values we use the growth function above to determine if our cell lives or dies. In this case U0 is the total value from all our neighbours combined and divided by the squared kernel size, and U1 is the same except only higher weights chosen.
After doing all of that, we get the following:
Whilst i was figuring all of this out, some interesting mistakes were made!
Here is a collection of either broken code, weird settings, or both!
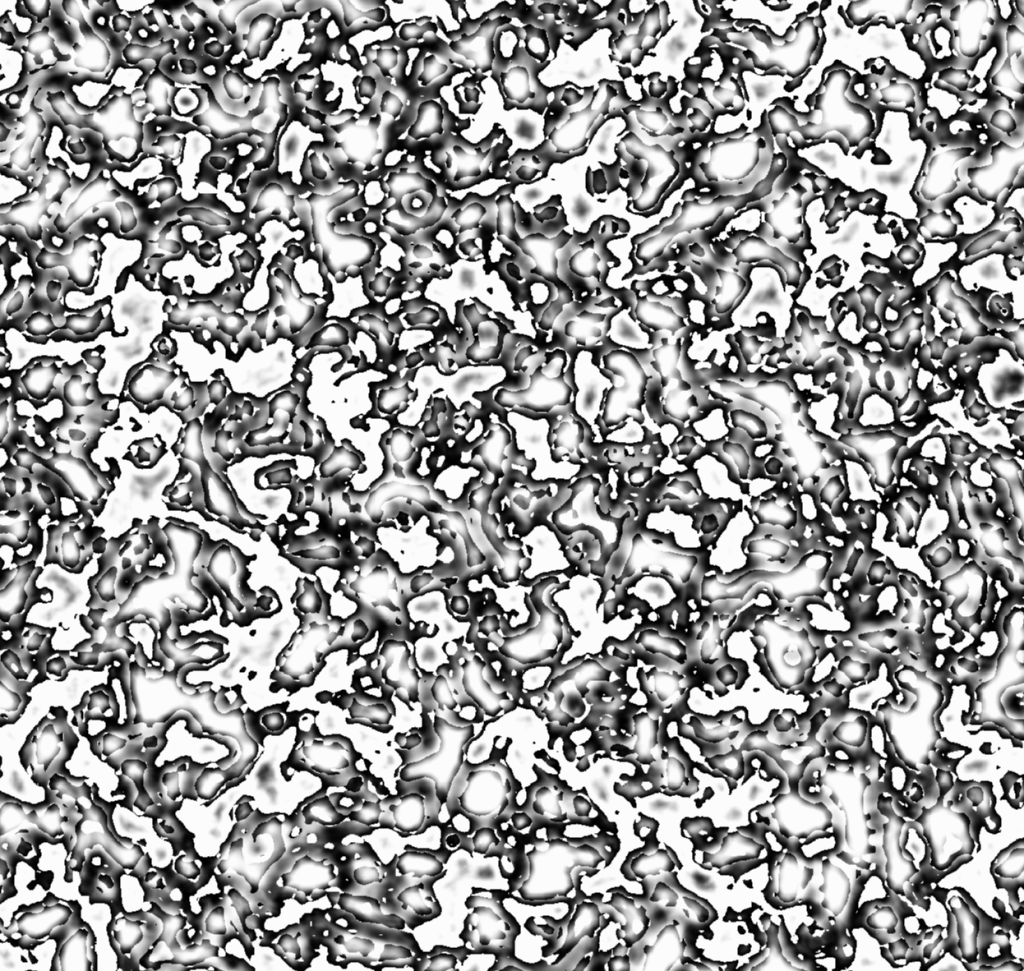
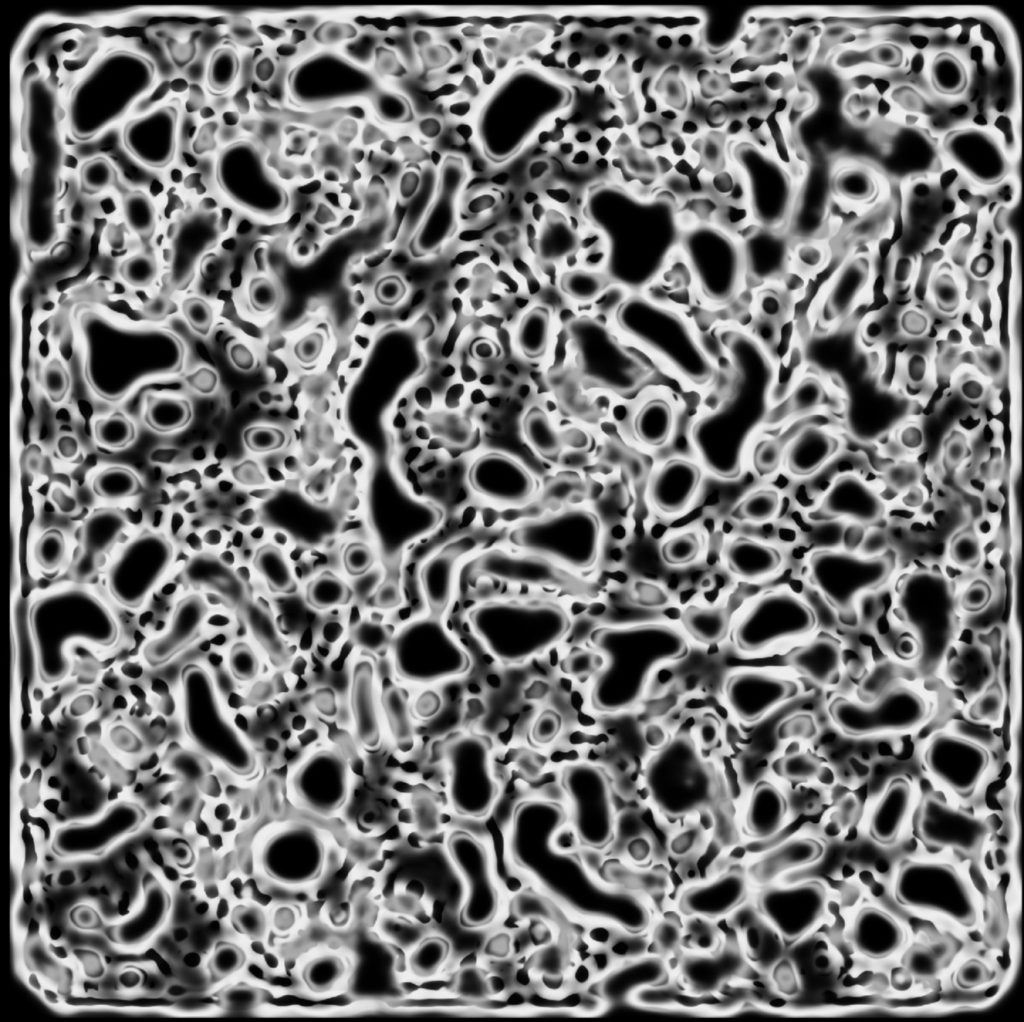
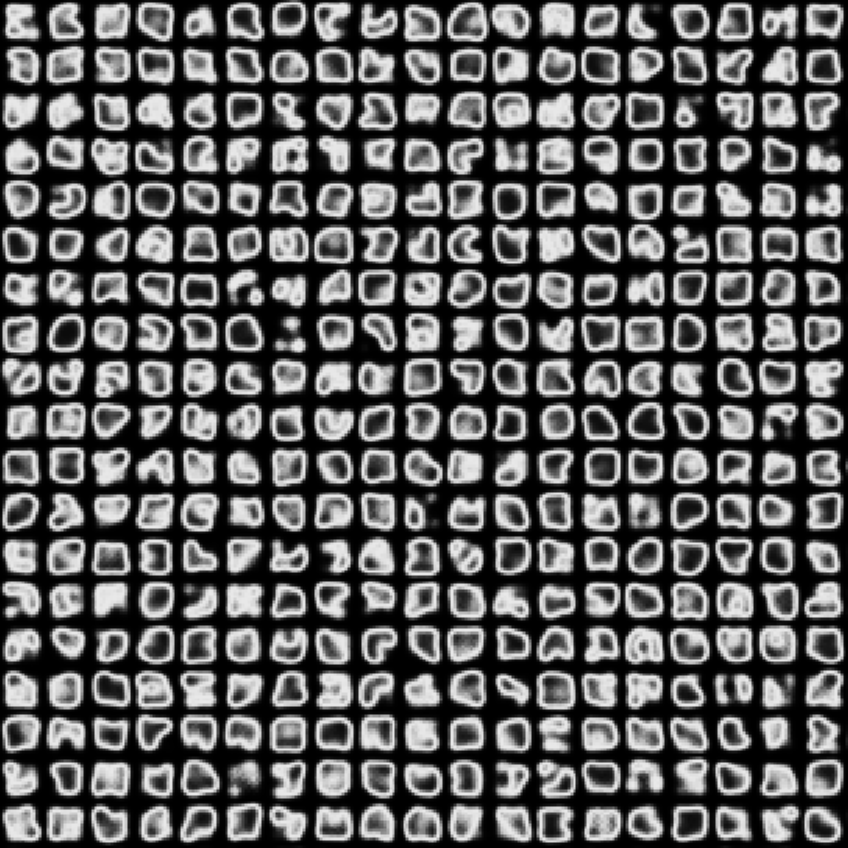
+ There are no comments
Add yours