This project is an SDF renderer made in Unity, using compute shaders and ray marching!
Ray marching is a similar technique to raytracing except it uses distance functions, and if its below a certain value (epsilon) it hit!
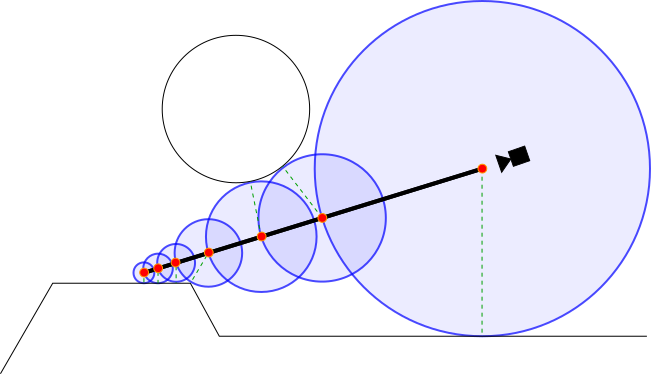
As you can see in the diagram above, you start at an origin, and then move in a certain direction, check how far away you are from something, and move to the edge of the sphere, check the nearest distance again, and if it’s below the epsilon, you hit something!
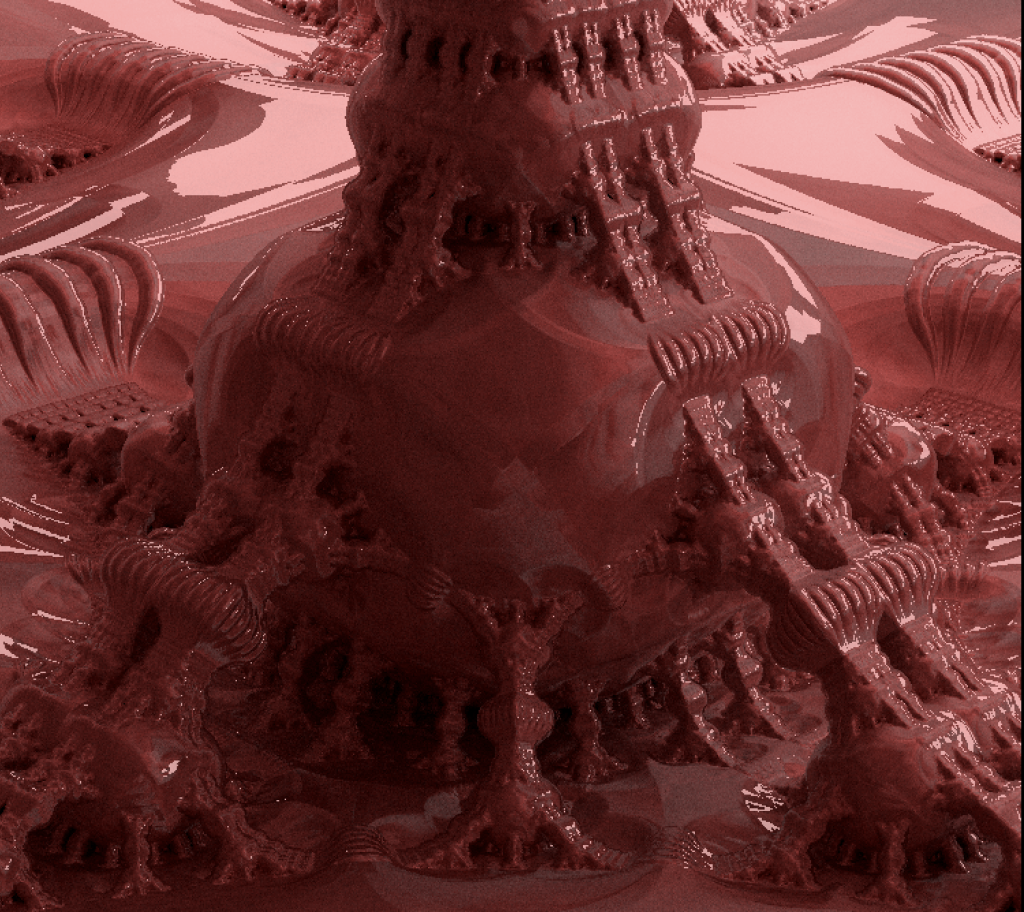
I also implemented subsurface scattering, speculars and diffuse! This allows for more realistic materials like flesh!
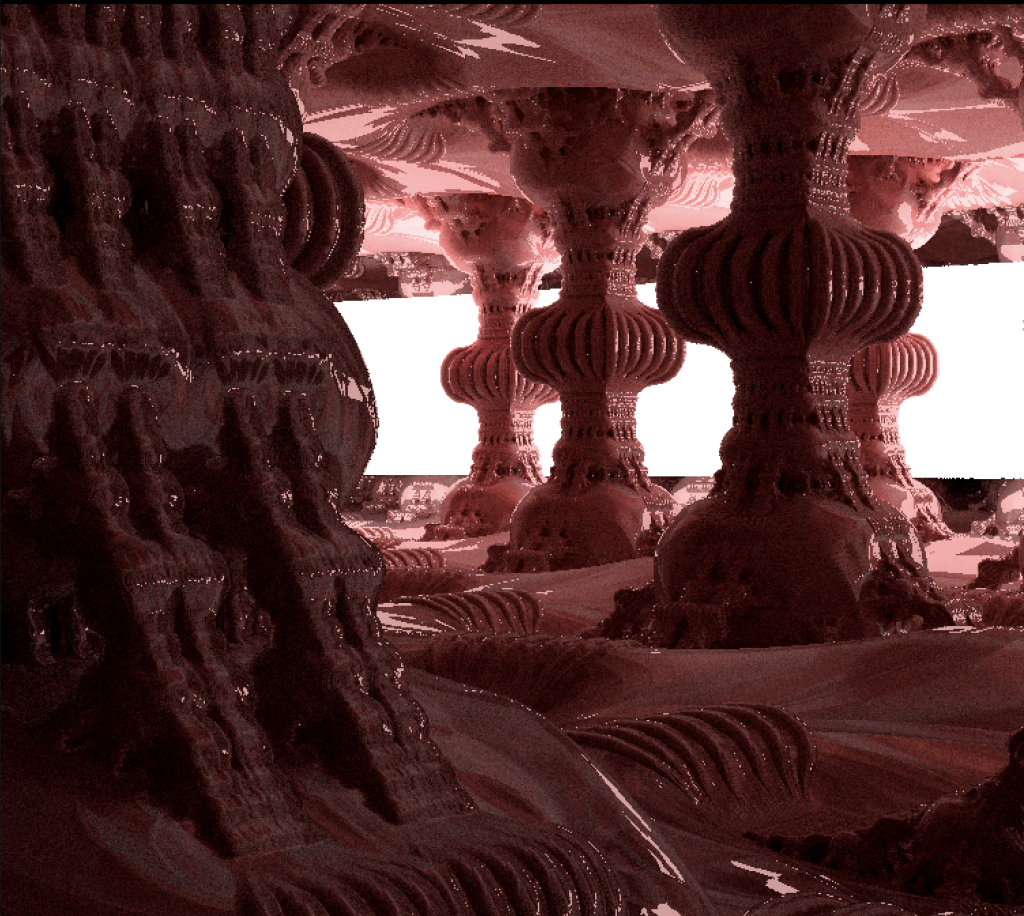
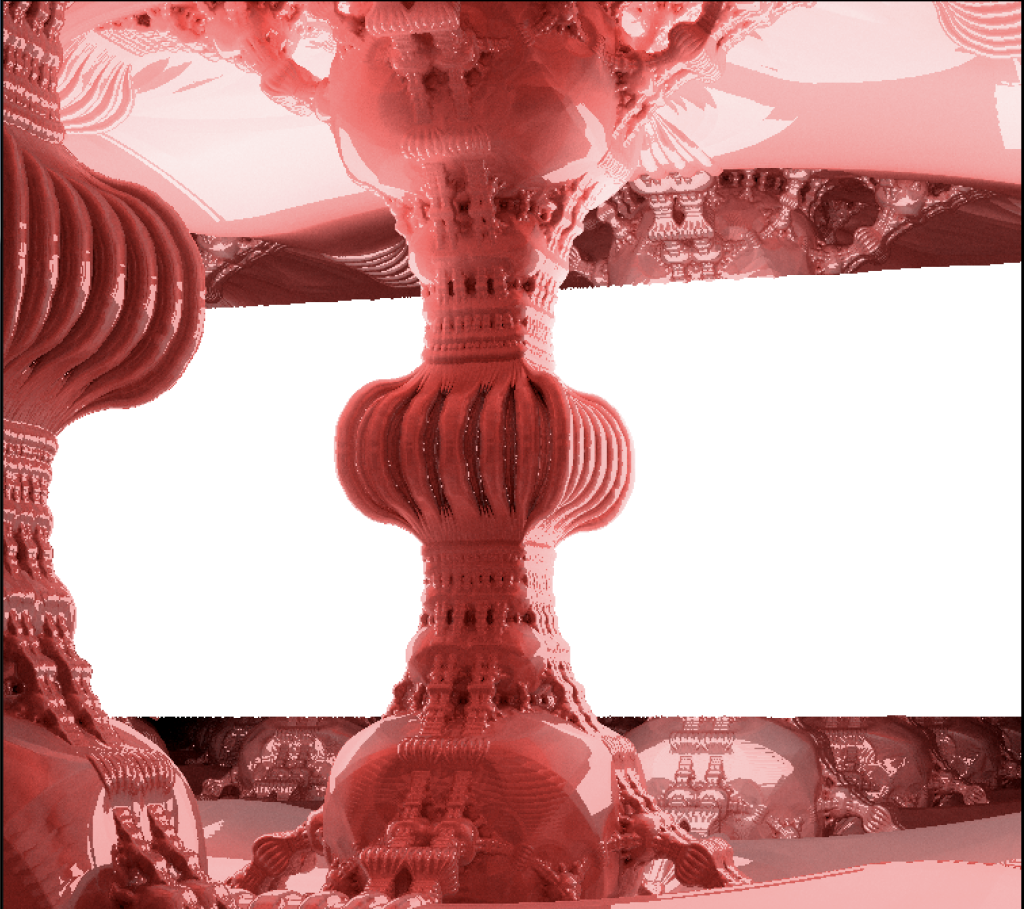
It supports ray traced (see above) rendering and regular rendering! This can be achieved by calculating normals for lighting calculations, which you can do by simply sampling the neighboring positions in space, and checking which are occupied, like so
float3 GetNormal(float3 p){
float3 e = float3(_epsilon, 0, 0);
return normalize(float3(
de(p + e.xyy) - de(p - e.xyy),
de(p + e.yxy) - de(p - e.yxy),
de(p + e.yyx) - de(p - e.yyx)
));
}
Where DE is a distance estimation function, in this case I found it on this awesome website: Distance Estimator Compendium (DEC) (jbaker.graphics)
Regular rendering implements AO (ambient occlusion), directional lighting and fog. These are all simple to implement, as fog is simply sampling the total distance from the origin, directional lighting is based on normals (no shadows though) and AO is checking the density of neighbors.
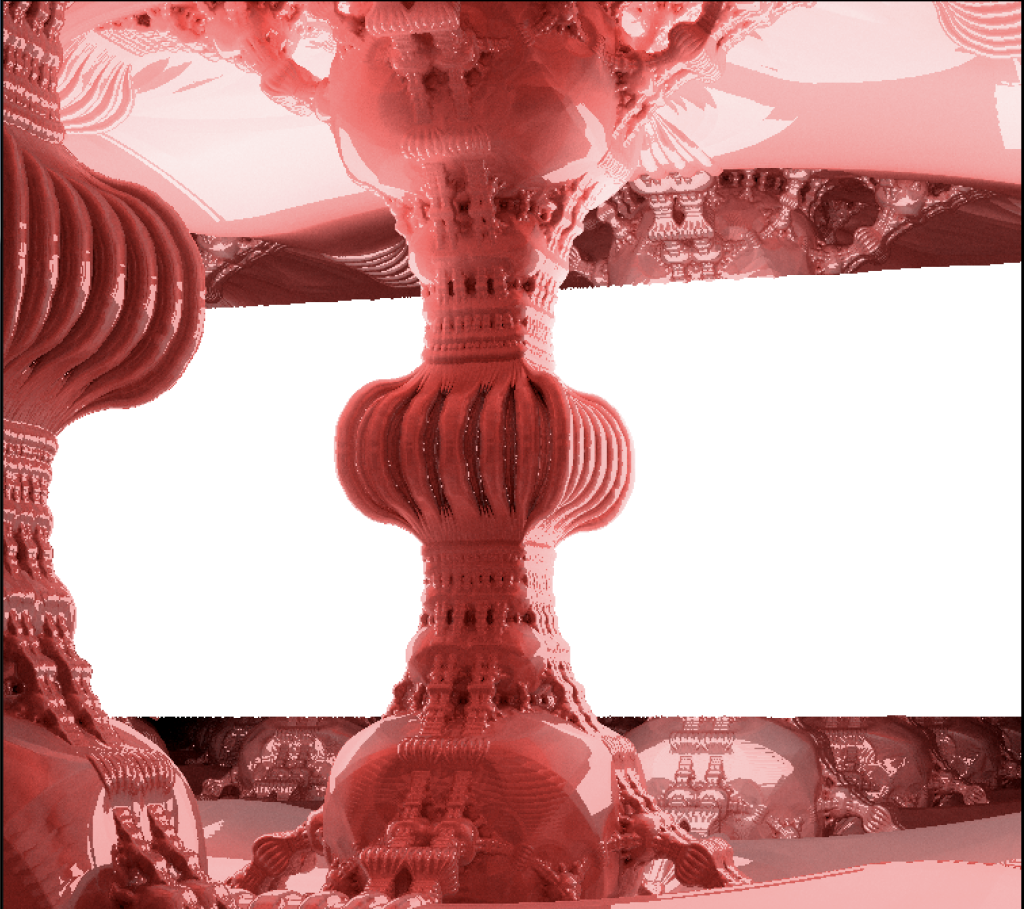
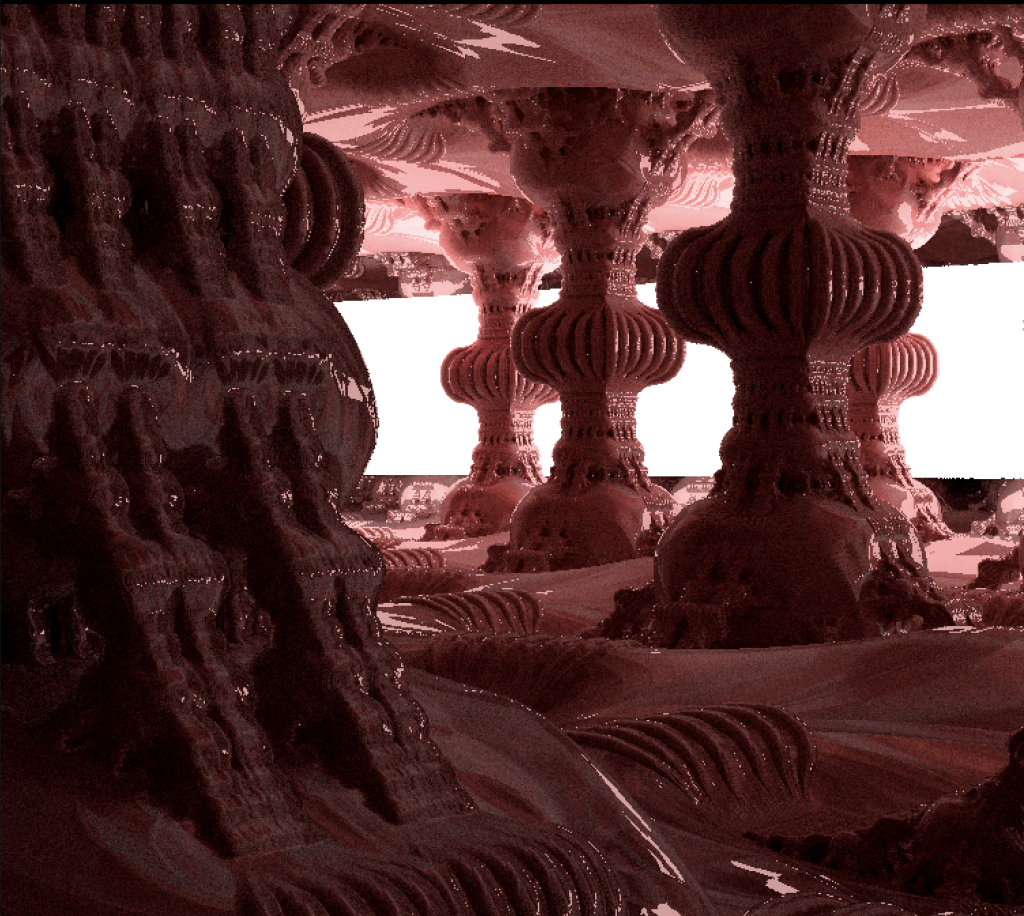
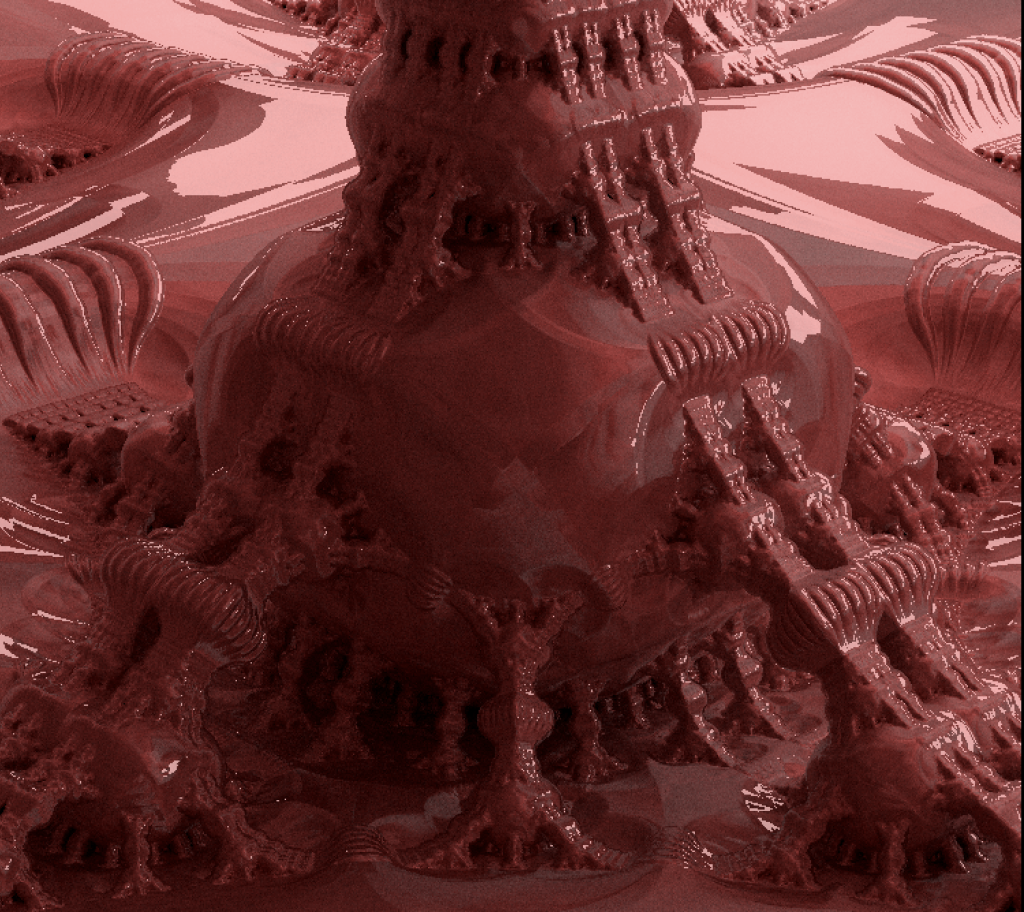
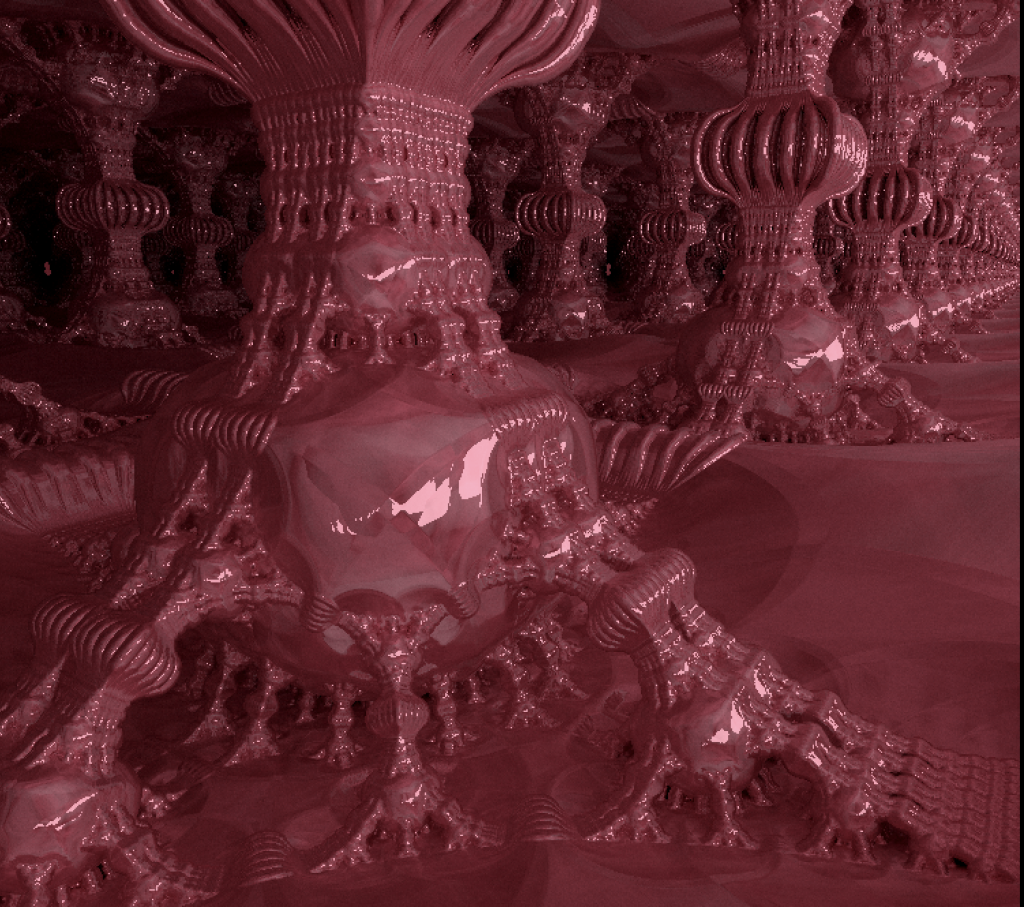
+ There are no comments
Add yours